You can find the Gist of movies references in this lesson here:
https://gist.github.com/tomgobich/2f29d392c67c9cc01dfa14e42f2995d5
Tapping into Model Factory States
In this lesson, we'll dive a little bit deeper into Model Factories by introducing factory states. We'll also learn how we can use the tap method to alter a factory result prior to it persisting into the database
- Author
- Tom Gobich
- Published
- Mar 15
- Duration
- 9m 15s
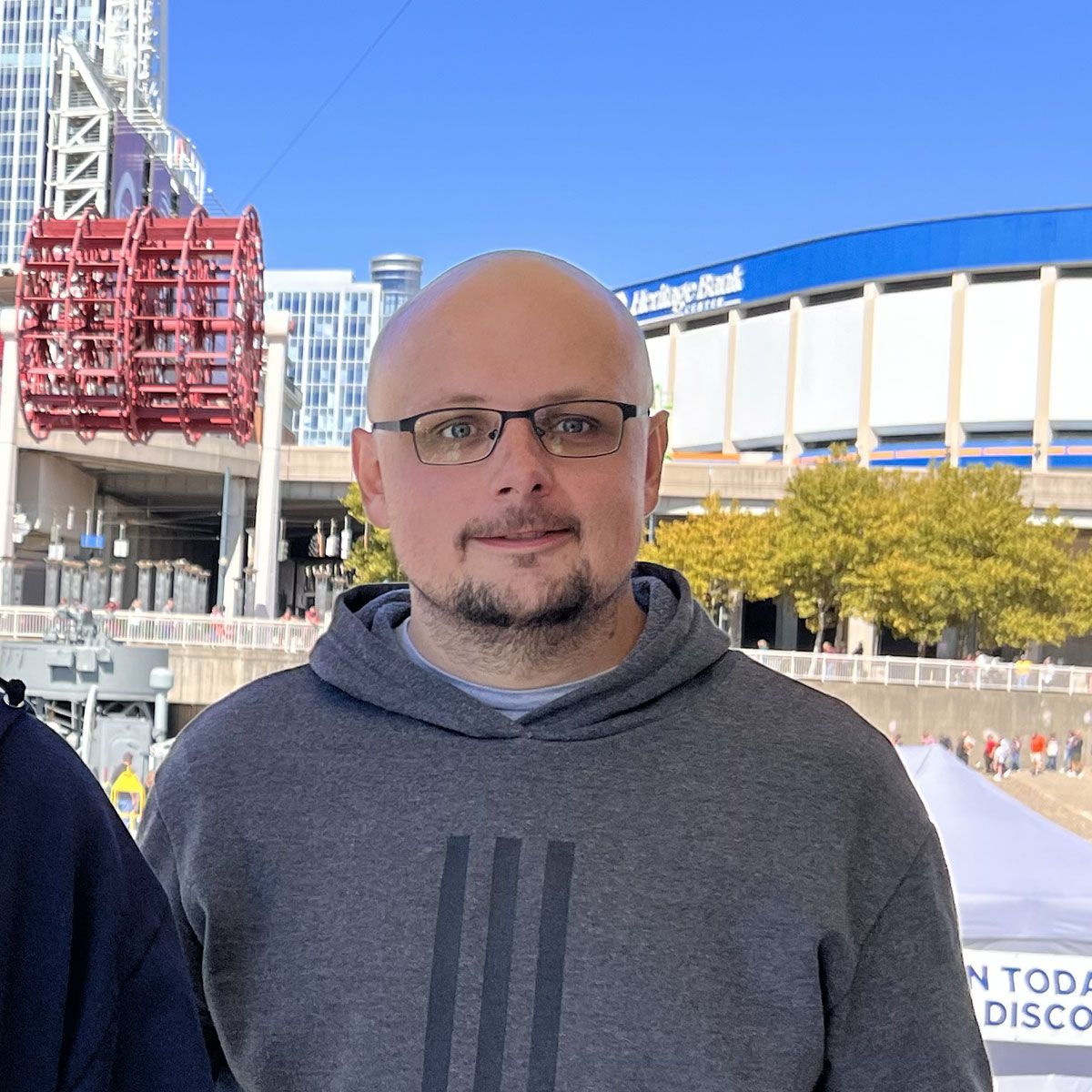
Developer, dog lover, and burrito eater. Currently teaching AdonisJS, a fully featured NodeJS framework, and running Adocasts where I post new lessons weekly. Professionally, I work with JavaScript, .Net C#, and SQL Server.
Adocasts
Burlington, KY
Transcript
Tapping into Model Factory States
-
(upbeat music)
-
So it'd be great if we actually had real movie titles
-
inside of our application using faker information
-
rather than just song names
-
because now our application feels more like a song database
-
rather than a movie database.
-
So I've taken movies listed from the National Film Registry
-
and I've normalized this into a JavaScript
-
or TypeScript array of objects containing a title
-
and a released year.
-
So today we're gonna take this list
-
and use it to seed our information
-
inside of our application.
-
So let's go ahead and hide our browser back away.
-
Now we could use this list of titles
-
directly inside of our Model Factory,
-
but it would be great to also be able to generate out
-
random movies that are future dated and the like
-
since our list stops at 2017.
-
So instead what we'll do is just use it
-
directly inside of our fake seeder.
-
It will just generate out one movie per record
-
that we have inside of this list.
-
Oops, I actually forgot to copy that list.
-
So let's dive back into this gist that I have here,
-
click on raw and let's Control or Command + A,
-
Control or Command + C to give that a copy.
-
Okay, now we can hide that back away.
-
And inside of our database directory,
-
let's go ahead and right click, new file,
-
and let's create a folder.
-
We could call this data, we could call this faker.
-
I'm just gonna call this data for now, movies.ts.
-
And let's go ahead and paste that list in
-
and give it a save so that it formats.
-
Cool, you see up here at line one,
-
we're exporting const movies.
-
So within our fake seeder, we can import that
-
and let's make use of it inside of a separate method
-
within here.
-
So we'll call this async, we'll make it private
-
and we'll call it create movies.
-
Okay, now we still wanna make use of our Movie Factory.
-
So we'll await movie factory.createMany
-
and we'll wanna create one movie record per movie
-
that we have inside of our data list here.
-
So we wanna import our movies, so we'll type out movies
-
and hit tab to auto import that from database data movies
-
and provide it its array length.
-
Now, currently this would create one movie
-
with any random song name as its title.
-
What we wanna do instead is tap into this
-
prior to its actual creation.
-
And with this tap method, we can provide a callback function
-
that's provided a row, which in this case
-
is going to be an instance of our movie model class.
-
It'll also provide us a factory context
-
and a factory builder.
-
So within here, we can do row,
-
provide that as a callback method
-
and now if we type out row,
-
we'll see that this looks exactly like a typical model would
-
so we can type out title and overwrite the title
-
prior to this record being persisted into the database
-
because tap is run just before it is persistent.
-
So within our tap callback method,
-
what we wanna do is grab the index
-
of the current movie that we're on.
-
So let's do let index equals zero.
-
We'll want to index plus plus at the end of this
-
to increment that.
-
We can go ahead and plop our movie into a variable.
-
So we'll do const movie equals movies.index
-
and then we can set the current movie title
-
by calling movie.title.
-
Now we can do something similar
-
with the release data as well.
-
Since we have the released year,
-
so we can use faker by extracting that
-
out of our factory context just like so.
-
We can do faker.date.
-
We can use the between method to start dates
-
that the random date should be between.
-
Since we only have the released year,
-
we can set this to a random date within that year
-
so that all of our movies aren't using the same month
-
and day as we're looping over them here.
-
So we'll say from and let's grab an instance
-
of a LuxonDateTime object set to the movies released year.
-
So we'll say released equals date time,
-
importing that from Luxon just like so.
-
So we'll say date time dot,
-
and we can just set this to now
-
and then overwrite the year to our movie.releasedYear.
-
Cool, so now this object is set to a LuxonDateTime
-
set to the current movies released year.
-
So meaning we can do released.startOf
-
to go to the start of the year
-
and then to released.endOf
-
to go to the end of the year.
-
Now we have a couple of issues here to fix.
-
Firstly, faker.date is going to return back
-
an actual JavaScript date,
-
whereas our released.dat is expecting a LuxonDateTime.
-
So we can wrap this in dateTime from JS date
-
to ingest the faker.date in as a LuxonDateTime.
-
Give that a save.
-
And now we have our second issue,
-
which is that faker in the from and to
-
is expecting a JavaScript date or a string or a number.
-
So we have a couple of options there.
-
We can either do toISO, toString, toFormat,
-
or we can just do toJSDate
-
to convert that to a JavaScript date.
-
We'll just go ahead with that one, cool.
-
So now as we loop through the movies that we're creating,
-
one per record inside of our movie array here,
-
we'll increment our index
-
so that we can get an instance of the movie
-
that we're currently on inside of our tap callback,
-
overwrite the record's title with the actual movie title,
-
and overwrite the released out with some random date
-
between January 1st and December 31st
-
of the current released year for that particular movie.
-
Before we give this a go,
-
let's go ahead and move our other movie factories,
-
creation calls inside of our createMovies
-
so that it's true to the method's name.
-
So we'll go ahead and plop these down at the end
-
after we run through our real movies, cool.
-
We can also clean this particular movie factory
-
up a little bit.
-
So instead of merging in
-
every time that we wanna create a movie that's released,
-
we can instead specify a state directly on the movie factory
-
and then just call that state here
-
so that we don't need to merge the state in
-
every single time.
-
So within our movie factory, we can scroll down here,
-
do .state, give the state a name.
-
So we could do released.
-
And then the second argument to this
-
is going to be very similar to the tap method.
-
It's going to provide us in the row for the movie
-
prior to it being persisted into the database.
-
And then we also get the factory context
-
and factory builder as well.
-
So we can extract faker out of this too.
-
So we can do pretty much the exact same thing
-
that we just did inside of our fake cedar
-
to specify a movie has been released
-
for whatever factory we're running this with.
-
So we could do row.statusId to set that to
-
movieStatuses.released,
-
and then row.releasedAt equals dateTime.fromJSDate.
-
We need to import that from Luxon.
-
So dateTime, hit tab to auto import that from Luxon,
-
just like so.
-
And then we can use faker.date.
-
And set this to sometime in the past
-
since the movie will have been released.
-
We can also do one for releasing soon.
-
So if we give that a copy and a paste,
-
we could do releasing soon,
-
or just soon if you want to keep that nice and short.
-
We can keep the movie status to released,
-
and this will give the scope that we defined
-
on our movie model a nice test case.
-
So we can set the release date to soon
-
rather than sometime in the past
-
so that our movie status is set to released,
-
but the release date is still sometime soon there
-
in the future.
-
So although the movie is set to released,
-
it's not actually physically released
-
because the release date has not been met yet.
-
Let's do one more.
-
So let's do one for post-production here.
-
So we'll do state name of post-production,
-
set the movie status to post-production,
-
and we can leave the faker date to soon there.
-
Okay, give that a save,
-
jump into our faker cedar,
-
and now we can replace this merge call here
-
instead by applying one of our states.
-
And for this, we just need to provide in the state name.
-
So here we were creating released movies,
-
so we'll call apply there with released as the argument,
-
and now we're creating our released movies
-
without having to merge in information for that.
-
We can give that a copy,
-
paste it two more times
-
because we also have releasing soon
-
as well as post-production that we can create as well.
-
So we give that a save.
-
Lastly, we need to actually call our create movie method.
-
So up here within our run method,
-
all we wanna do is await this create movies, just like so.
-
Awesome.
-
Now we're ready to go ahead and roll back our database,
-
rerun everything and reseed our database as well.
-
Now, one thing to note before we do that though,
-
is that you don't wanna place this data directory
-
inside of cedars because whenever we run our seed command,
-
Athonis JS is going to try and auto import
-
whatever cedar files we have within here,
-
and if it's not an actual cedar,
-
it's going to result in an error.
-
So we've placed our data directory
-
directly underneath database here
-
for a very particular reason,
-
even though we're only using it inside of our cedars.
-
Okay, so let's go ahead and hide our text editor back away.
-
Let's reset our database.
-
So node ace migration reset.
-
This is going to roll back
-
all of the migrations that we have,
-
regardless of what batch number it was run within.
-
Okay, let's go ahead and node ace migration run
-
to rerun those migrations.
-
All right, and lastly, let's go ahead and node ace db seed.
-
And we've run into an error.
-
Now, here it's saying it cannot insert into users
-
and specifically it's complaining
-
about the user's role ID foreign key constraint.
-
Essentially, we're trying to insert into users
-
because our fake cedar is running prior to our start cedar.
-
So our roles don't actually exist
-
at the time that we're trying to insert
-
into our user's database.
-
So what we need to do is specify
-
that we want the start cedar to run before our fake cedar,
-
so that those roles and the information
-
that we're creating inside of our start cedar
-
actually exists whenever we get to our fake cedar.
-
So let's go ahead and node ace migration reset
-
to roll back all of our database migrations
-
because our start cedar did succeed.
-
So we wanna get rid of that information
-
to test to make sure that our fix here works properly.
-
Dive back in the Visual Studio Code.
-
And by default, AdonisJS is going to run these
-
similar to migrations.
-
It's going to run them in alphabetical order
-
or whatever order they're displayed in
-
using a natural sort.
-
And we want our fake cedar to run after our start cedar.
-
So we'll just do 01 with an underscore there.
-
And now we'll jump down to our start cedar.
-
And we're always going to want this to run first.
-
So we'll do 00.
-
So now our start cedar is naturally sorted
-
before our fake cedar.
-
So AdonisJS should now run them
-
in the order that we want them to.
-
So we can hide our text editor back away.
-
We've already reset our database.
-
So let's go ahead and re-migrate it.
-
So node is migration run.
-
Cool, let's clear that out.
-
And let's go ahead and seed our database once more.
-
There we go, awesome.
-
So it ran it in the order that we wanted it to
-
and everything completed successfully.
-
All right, let's go and run our server.
-
So npm run dev, jump back into our browser,
-
jump over to our application and look at that.
-
Wow, we can keep scrolling and scrolling and scrolling
-
and we're just gonna have a bunch of movies
-
and even better yet, we now have actual movie titles.
-
Some of these are gonna be song names,
-
but for the most part, what we have here
-
are actual movie titles that we're working with now.
-
Awesome.
-
So now we need to take care of the number
-
that we're displaying,
-
but we have plenty of movies to work with now
-
at our disposal.
-
Introduction
-
Fundamentals
-
2.0Routes and How To Create Them5m 23s
-
2.1Rendering a View for a Route6m 29s
-
2.2Linking Between Routes7m 51s
-
2.3Loading A Movie Using Route Parameters9m 17s
-
2.4Validating Route Parameters6m 6s
-
2.5Vite and Our Assets6m 38s
-
2.6Setting Up Tailwind CSS9m 5s
-
2.7Reading and Supporting Markdown Content4m 32s
-
2.8Listing Movies from their Markdown Files8m 51s
-
2.9Extracting Reusable Code with Services7m 4s
-
2.10Cleaning Up Routes with Controllers4m 52s
-
2.11Defining A Structure for our Movie using Models9m 38s
-
2.12Singleton Services and the Idea of Caching6m 11s
-
2.13Environment Variables and their Validation4m 16s
-
2.14Improved Caching with Redis10m 44s
-
2.15Deleting Items and Flushing our Redis Cache6m 46s
-
2.16Quick Start Apps with Custom Starter Kits6m 28s
-
2.17Easy Imports with NodeJS Subpath Imports8m 40s
-
-
Building Views with EdgeJS
-
3.0EdgeJS Templating Basics8m 49s
-
3.1HTML Attribute and Class Utilities6m 9s
-
3.2Making A Reusable Movie Card Component10m 24s
-
3.3Component Tags, State, and Props4m 53s
-
3.4Use Slots To Make A Button Component6m 56s
-
3.5Extracting A Layout Component5m 13s
-
3.6State vs Share Data Flow2m 59s
-
3.7Share vs Global Data Flow6m 7s
-
3.8Form Basics and CSRF Protection6m 13s
-
3.9HTTP Method Spoofing HTML Forms3m 3s
-
3.10Easy SVG Icons with Edge Iconify7m 57s
-
-
Database and Lucid ORM Basics
-
4.0Configuring Lucid and our Database Connection4m 3s
-
4.1Understanding our Database Schema9m 35s
-
4.2Introducing and Defining Database Migrations18m 35s
-
4.3The Flow of Migrations8m 28s
-
4.4Introducing Lucid Models5m 43s
-
4.5Defining Our Models6m 49s
-
4.6The Basics of CRUD11m 56s
-
4.7Defining Required Data with Seeders11m 11s
-
4.8Stubbing Fake Data with Model Factories13m 48s
-
4.9Querying Our Movies with the Query Builder15m 30s
-
4.10Unmapped and Computed Model Properties3m 24s
-
4.11Altering Tables with Migrations7m 6s
-
4.12Adding A Profile Model, Migration, Factory, and Controller2m 57s
-
4.13SQL Parameters and Injection Protection9m 19s
-
4.14Reusable Query Statements with Model Query Scopes8m 11s
-
4.15Tapping into Model Factory States9m 15s
-
4.16Querying Recently Released and Coming Soon Movies4m 59s
-
4.17Generating A Unique Movie Slug With Model Hooks7m 59s
-
-
Lucid ORM Relationships
-
5.0Defining One to One Relationships Within Lucid Models5m 49s
-
5.1Model Factory Relationships2m 54s
-
5.2Querying Relationships and Eager Vs Lazy Loading5m 17s
-
5.3Cascading and Deleting Model Relationships5m 16s
-
5.4Defining One to Many Relationships with Lucid Models6m 56s
-
5.5Seeding Movies with One to Many Model Factory Relationships5m 24s
-
5.6Listing A Director's Movies with Relationship Existence Queries8m 41s
-
5.7Listing and Counting a Writer's Movies8m 41s
-
5.8Using Eager and Lazy Loading to Load A Movie's Writer and Director5m 18s
-
5.9Defining Many-To-Many Relationships and Pivot Columns9m 48s
-
5.10Many-To-Many Model Factory Relationships4m 50s
-
5.11A Deep Dive Into Relationship CRUD with Models18m 5s
-
5.12How To Create Factory Relationships from a Pool of Data13m 55s
-
5.13How To Query, Sort, and Filter by Pivot Table Data9m 47s
-
-
Working With Forms
-
6.0Accepting Form Data12m 15s
-
6.1Validating Form Data with VineJS9m 29s
-
6.2Displaying Validation Errors and Validating from our Request7m 16s
-
6.3Reusing Old Form Values After A Validation Error2m 3s
-
6.4Creating An EdgeJS Form Input Component5m 28s
-
6.5Creating A Login Form and Validator5m 1s
-
6.6How To Create A Custom VineJS Validation Rule9m 7s
-
-
Authentication & Middleware
-
The Flow of Middleware7m 49s
-
Authenticating A Newly Registered User4m 14s
-
Checking For and Populating an Authenticated User2m 10s
-
Logging Out An Authenticated User2m 24s
-
Logging In An Existing User6m 54s
-
Remembering A User's Authenticated Session6m 55s
-
Protecting Routes with Auth, Guest, and Admin Middleware5m 36s
-
-
Filtering and Paginating Queries
Join The Discussion! (0 Comments)
Please sign in or sign up for free to join in on the dicussion.
Be the first to Comment!